Last Updated on August 18, 2024 by Max
Single-slit diffraction is a fundamental phenomenon in matter wave optics, illustrating the wave nature of particles such as electrons. In this article, we present an animation of single-slit diffraction of electrons using the split-step Fourier method.
The simulation captures the evolution of the wave packet as it interacts with a slit, showcasing the resulting diffraction pattern. This visualization serves as a powerful tool for understanding quantum behavior and the principles of wave-particle duality clearly and intuitively. The accompanying code provides a detailed implementation of the process.
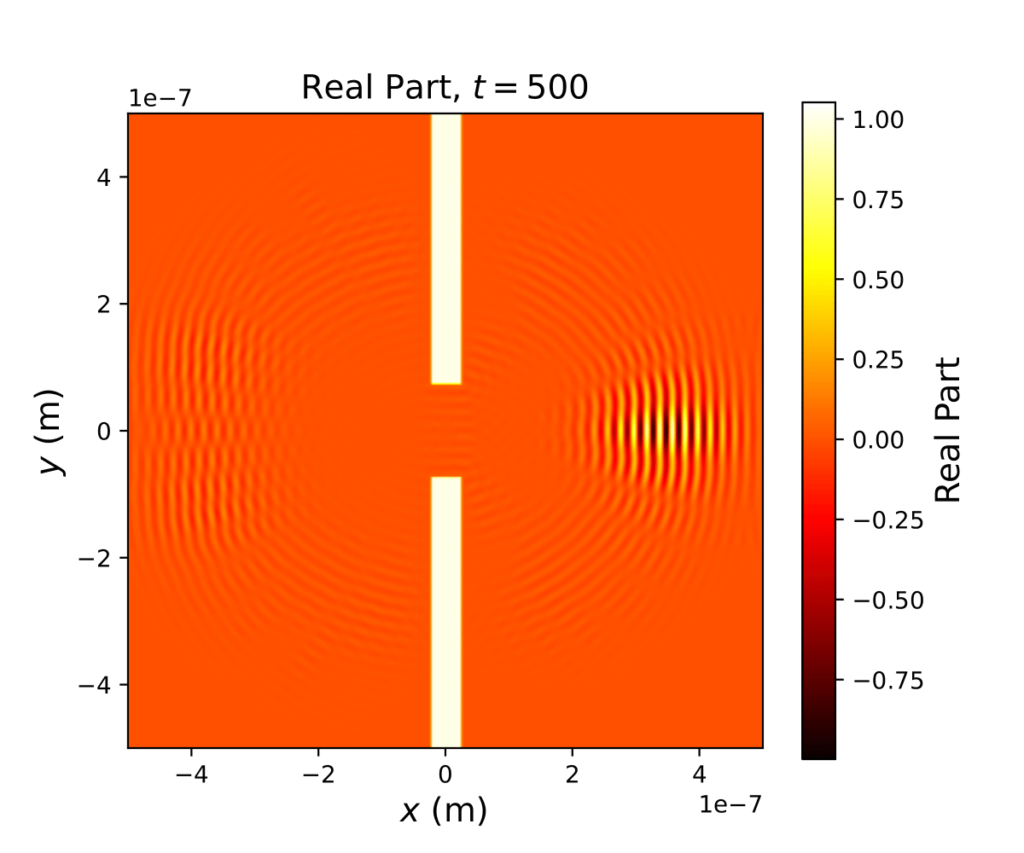
Numerical Method
Initial Setup and Parameters
- Reduced Planck Constant: \( \hbar = 1.0545718 \times 10^{-34} \) J·s.
- Mass of Electron: \( m_e = 9.10938356 \times 10^{-31} \) kg.
- de Broglie Wavelength: Given as 20 nm (\( \lambda = 20 \times 10^{-9} \) m).
- Wave Number: \( k_0 = \frac{2\pi}{\lambda} \)
Grid and Simulation Domain
- The simulation domain size is set to \( L_x = L_y = 1 \times 10^{-6} \) meters with grid points \( N_x = N_y = 512 \).
- The grid spacing: \( dx = \frac{L_x}{N_x}, \quad dy = \frac{L_y}{N_y} \)
- The mesh grid \((X, Y)\) is created to represent spatial coordinates in the domain.
Initial Gaussian Wave Packet
The initial wave packet is modeled as a Gaussian function in both \(x\) and \(y\) directions with a plane wave factor,
\[\psi_0(x, y) = \exp\left(-\frac{(X – x_0)^2}{2\sigma_x^2} – \frac{(Y – y_0)^2}{2\sigma_y^2}\right) \times \exp\left(i(k_{x0} X + k_{y0} Y)\right).\]
Normalization: The wave packet is normalized to ensure the total probability density is 1 : \( \psi_0 \div \sqrt{\sum|\psi_0|^2}. \)
Potential for Single Slit
The potential barrier \(V\) is defined as a slit of width 150 nm centered in the domain. The potential is set to a high value (\(V_0 = 1 \times 10^{10}\) J) in the regions outside the slit.
Fourier Space Components
Wave Numbers (\(K_x, K_y\)): Calculated using the `fft` frequencies:
\[ K_x = \frac{2\pi}{dx \times N_x}, \quad K_y = \frac{2\pi}{dy \times N_y} \]
Square of the Wave Numbers: \( K^2 = K_x^2 + K_y^2 \)
Split-Step Fourier Method
The time evolution of the wave packet is simulated using the split-step Fourier method, which involves the following steps:
1. Half-Step Evolution in Real Space (Potential Energy Evolution):
\[ \psi(x, y) \times \exp\left(-\frac{i V(x, y) \Delta t}{2\hbar}\right) \]
2. Full-Step Evolution in Fourier Space (Kinetic Energy Evolution):
- Apply Fourier transform to move to Fourier space: \( \psi_k = \mathcal{F}\{\psi(x, y)\} \)
- Evolve the wave function in Fourier space:
\[ \psi_k \times \exp\left(-\frac{i \hbar K^2 \Delta t}{2m_e}\right) \]
- Apply inverse Fourier transform to return to real space:
\[ \psi(x, y) = \mathcal{F}^{-1}\{\psi_k\} \]
3. Another Half-Step Evolution in Real Space (Potential Energy Evolution):
\[ \psi(x, y) \times \exp\left(-\frac{i V(x, y) \Delta t}{2\hbar}\right) \]
Probability Density and Animation
- The probability density \((\rho)\) is computed as: \( \rho(x, y) = |\psi(x, y)|^2 \)
- The real and imaginary parts of the wave function are also computed and visualized:
\[ \text{Real Part} = \text{Re}(\psi(x, y)), \quad \text{Imaginary Part} = \text{Im}(\psi(x, y)) \]
- An animation is created by updating these quantities over time steps.
This step-by-step approach allows for the simulation of how an electron’s wave packet evolves and diffracts as it passes through a single slit, producing a diffraction pattern characteristic of wave behavior.
Animation Code in Python
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.animation as animation
# Constants
hbar = 1.0545718e-34 # Reduced Planck constant (Joule second)
m_e = 9.10938356e-31 # Mass of electron (kg)
wavelength = 20e-9 # de Broglie wavelength (meters)
k0 = 2 * np.pi / wavelength # Wave number
# Grid parameters
Lx, Ly = 1e-6, 1e-6 # Simulation domain size (meters)
Nx, Ny = 512, 512 # Number of grid points
dx, dy = Lx / Nx, Ly / Ny # Grid spacing
x = np.linspace(-Lx / 2, Lx / 2, Nx)
y = np.linspace(-Ly / 2, Ly / 2, Ny)
X, Y = np.meshgrid(x, y)
# Time parameters
dt = 3e-14 # Time step (seconds)
Nt = 501 # Number of time steps
# Wave packet parameters
sigma_x = sigma_y = 60e-9 # Width of the Gaussian packet (meters)
x0, y0 = -2e-7, 0 # Initial position of the packet (meters)
kx0, ky0 = k0, 0 # Initial wave vector
# Initial Gaussian wave packet
psi0 = np.exp(-(X - x0) ** 2 / (2 * sigma_x ** 2)) * np.exp(-(Y - y0) ** 2 / (2 * sigma_y ** 2))
psi0 = psi0.astype(np.complex128)
psi0 *= np.exp(1j * (kx0 * X + ky0 * Y))
# Normalize the initial wave packet
psi0 /= np.sqrt(np.sum(np.abs(psi0) ** 2))
# Potential for single slit
slit_width = 150e-9 # Width of the slit (meters)
wall_thickness = 50e-9 # Thickness of the slit walls (meters)
V0 = 1e10 # Potential barrier height (Joules)
V = np.zeros((Nx, Ny)) # Initialize the potential to zero
# Define the slit region
x1 = int(Nx / 2) - int(0.5 * wall_thickness / dx)
x2 = int(Nx / 2) + int(0.5 * wall_thickness / dx)
for i in range(x1, x2):
for j in range(Ny):
if j <= (int(0.5 * Ny) - int(0.5 * slit_width / dy)) or j >= (int(0.5 * Ny) + int(0.5 * slit_width / dy)):
V[j, i] = V0
# Fourier space components
kx = np.fft.fftfreq(Nx, dx) * 2 * np.pi
ky = np.fft.fftfreq(Ny, dy) * 2 * np.pi
KX, KY = np.meshgrid(kx, ky)
K2 = KX ** 2 + KY ** 2
# Split-step Fourier method
psi = psi0.copy()
# Prepare for animation
fig, axs = plt.subplots(3, 1, figsize=(6, 15))
# Plot probability density (Rho)
Rho = np.abs(psi) ** 2
im1 = axs[0].imshow(Rho / Rho.max() + V / V0, extent=(-Lx / 2, Lx / 2, -Ly / 2, Ly / 2), cmap='hot', animated=True)
cbar1 = fig.colorbar(im1, ax=axs[0], orientation='vertical')
cbar1.set_label('Probability Density')
axs[0].set_xlabel(r'$x$ (m)', fontsize=14)
axs[0].set_ylabel(r'$y$ (m)', fontsize=14)
# Plot real part of the wave packet
real_part = np.real(psi)
im2 = axs[1].imshow(real_part / real_part.max() + V / V0, extent=(-Lx / 2, Lx / 2, -Ly / 2, Ly / 2), cmap='hot', animated=True)
cbar2 = fig.colorbar(im2, ax=axs[1], orientation='vertical')
cbar2.set_label('Real Part')
axs[1].set_xlabel(r'$x$ (m)', fontsize=14)
axs[1].set_ylabel(r'$y$ (m)', fontsize=14)
# Plot imaginary part of the wave packet
imag_part = np.imag(psi)
im3 = axs[2].imshow(imag_part / imag_part.max() + V / V0, extent=(-Lx / 2, Lx / 2, -Ly / 2, Ly / 2), cmap='hot', animated=True)
cbar3 = fig.colorbar(im3, ax=axs[2], orientation='vertical')
cbar3.set_label('Imaginary Part')
axs[2].set_xlabel(r'$x$ (m)', fontsize=14)
axs[2].set_ylabel(r'$y$ (m)', fontsize=14)
def update(t):
global psi
# (a) 1/2 Evolution for the potential energy in real space
psi *= np.exp(-1j * V * dt / (2 * hbar))
# (b) Forward transform
psi_k = np.fft.fft2(psi)
# (c) Full evolution for the kinetic energy in Fourier space
psi_k *= np.exp(-1j * hbar * K2 * dt / (2 * m_e))
# (d) Inverse Fourier transform
psi = np.fft.ifft2(psi_k)
# (e) 1/2 Evolution for the potential energy in real space
psi *= np.exp(-1j * V * dt / (2 * hbar))
# Update plots
Rho = np.abs(psi) ** 2
im1.set_array(Rho / Rho.max() + V / V0)
axs[0].set_title(f'Time step: {t}', fontsize=14)
real_part = np.real(psi)
im2.set_array(real_part / real_part.max() + V / V0)
imag_part = np.imag(psi)
im3.set_array(imag_part / imag_part.max() + V / V0)
return [im1, im2, im3]
ani = animation.FuncAnimation(fig, update, frames=Nt, repeat=False)
# Save the animation
ani.save('single_slit_diffraction_animation.mp4', writer='ffmpeg', fps=20)
plt.show()
The following three packages are needed to run the code:
- NumPy
- Matplotlib
- FFmpeg
Make sure that these packages are installed on the computer before running the code.
Animation Results
The simulation illustrates the wave nature of electrons as they pass through a single slit, resulting in a diffraction pattern. When the electron wave packet encounters the slit, it experiences spatial confinement, causing the wave to spread out as it passes through. This spreading leads to a diffraction pattern, visible in the probability density plot. The plot shows a central bright fringe, where most electrons are detected, surrounded by alternating dark and bright fringes. These fringes represent areas of destructive and constructive interference, respectively.
The real and imaginary parts of the wave function show an oscillatory pattern, reflecting the phase changes across the wavefronts. This behavior confirms the quantum mechanical principle that particles, such as electrons, exhibit wave-like properties. The width and spacing of the diffraction fringes depend on both the slit width and the electron’s de Broglie wavelength. The simulation clearly visualizes this fundamental concept, making it easier to understand.
Summary
This article presents a Python simulation of single-slit diffraction of electrons, illustrating the wave nature of particles through the split-step Fourier method.
The simulation captures the evolution of an electron wave packet as it passes through a slit, resulting in a characteristic diffraction pattern. The article provides detailed explanations of the numerical methods used, including the initialization of the Gaussian wave packet, the application of potential barriers, and the time evolution using Fourier transforms.
The results visually confirm quantum mechanical principles, showcasing the wave-particle duality and the interference patterns formed by electrons.

I am a science enthusiast and writer, specializing in matter-wave optics and related technologies. My goal is to promote awareness and understanding of these advanced fields among students and the general public.