Last Updated on November 30, 2024 by Max
In this comprehensive guide, we explore the power of Python to create high-quality plots essential for research. From basic plotting techniques to advanced customization using Matplotlib and NumPy, this article provides researchers with the tools to visualize data effectively, enhancing clarity and impact in scientific communication. Whether you’re a beginner or an expert, master Python plots easily.
A simple plot in Python
Here, we plot a parabolic function, \(y = x^2\), using Python and Matplotlib. The plot employs LaTeX-style fonts to enhance the clarity and quality of the text elements. The NumPy library is used for numerical calculations, while Matplotlib provides powerful tools for creating and customizing the plot.

Python Code
#### IMPORT LIBRARIES
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.pyplot import rcParams as r
#### FRAME PROPERTIES
r["figure.figsize"] = (2.8, 2.5) # Ensure figsize is a tuple
plt.rc("axes", linewidth=1)
# Enable LaTeX font style for all texts
plt.rcParams['text.usetex'] = True
plt.rcParams['font.family'] = 'serif'
plt.rcParams['font.serif'] = ['Computer Modern']
# Generate data for a parabolic function
x_data = np.linspace(-10, 10, 20) # 20 x-points from -10 to 10
y_data = x_data**2 # Parabolic function y = x^2
# Create a single-axis plot
fig, ax = plt.subplots()
# Plot the parabolic function
ax.plot(x_data, y_data, '-->b', ms=7, lw=1.5, label=r"$y = x^2$")
# Set axis properties
ax.tick_params(which="both", direction="in", top=True, right=True, length=4, width=1, labelsize=11)
# Add legends
ax.legend(loc='upper center', fontsize=14)
# Axes labels
ax.set_ylabel(r"$y$", fontsize=14)
ax.set_xlabel(r"$x$", fontsize=14)
# Axes ticks
ax.set_xticks([-10, -5, 0, 5, 10])
ax.set_yticks([0, 25, 50, 75, 100])
# Adjust layout and display the plot
plt.tight_layout()
plt.show()
Scatter Plot
A scatter plot is a visual tool used to represent individual data points defined by two variables, typically plotted along the x-axis and y-axis. Each point corresponds to a specific pair of values, enabling the visualization of relationships or trends between the variables.
In scientific research, scatter plots are essential for analyzing correlations, uncovering patterns, and identifying anomalies within datasets.
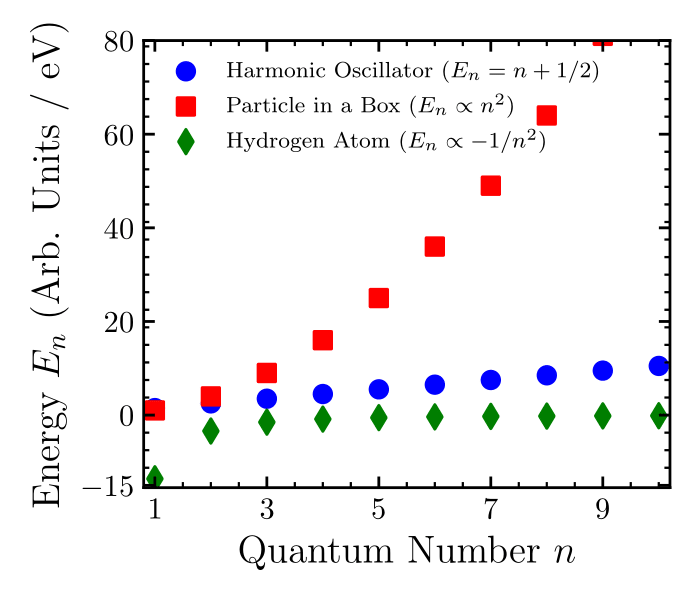
Python code:
The code below generates a scatter plot comparing energy eigenvalues for three quantum systems: a quantum harmonic oscillator (\(E_n = n + 1/2\)), a particle in a 1D box (\(E_n \propto n^2\)), and a hydrogen atom (\(E_n \propto -1/n^2\)). It uses scientific styling, LaTeX labels, and optimized axis limits for clear visualization.
#### IMPORT LIBRARIES
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.pyplot import rcParams as r
#### FRAME PROPERTIES
r["figure.figsize"] = (3.5, 3) # Ensure figsize is a tuple
plt.rc("axes", linewidth=1)
# Enable LaTeX font style for all texts
plt.rcParams['text.usetex'] = True
plt.rcParams['font.family'] = 'serif'
plt.rcParams['font.serif'] = ['Computer Modern']
# Generate data for three quantum systems
quantum_numbers = np.arange(1, 11) # Quantum numbers n = 1 to 10
# Quantum Harmonic Oscillator
E_harmonic = quantum_numbers + 0.5 # E_n = (n + 1/2) in arbitrary units
# Particle in a 1D Box
E_box = quantum_numbers**2 # E_n proportional to n^2 (arbitrary units)
# Hydrogen Atom
E_hydrogen = -13.6 / quantum_numbers**2 # Energy in eV
# Create a single-axis plot
fig, ax = plt.subplots()
# Plot the energy eigenvalues using scatter plot
ax.scatter(quantum_numbers, E_harmonic, c='blue', marker='o', s=40, label=r"Harmonic Oscillator ($E_n = n + 1/2$)")
ax.scatter(quantum_numbers, E_box, c='red', marker='s', s=40, label=r"Particle in a Box ($E_n \propto n^2$)")
ax.scatter(quantum_numbers, E_hydrogen, c='green', marker='d', s=40, label=r"Hydrogen Atom ($E_n \propto -1/n^2$)")
# Set axis properties
ax.tick_params(which="both", direction="in", top=True, right=True, length=4, width=1, labelsize=11)
# Axes labels
ax.set_ylabel(r"Energy $E_n$ (Arb. Units / eV)", fontsize=14)
ax.set_xlabel(r"Quantum Number $n$", fontsize=14)
# Adjust axes limits for scientific aesthetics
ax.set_xlim(0.8, 10.2) # Add a small buffer around quantum number range
ax.set_ylim(-15.5, 80) # Adjust y-axis for better visualization of all data
# Adjust tick intervals and set minor ticks
ax.set_xticks([1, 3, 5, 7, 9])
ax.set_yticks([-15, 0, 20, 40, 60, 80])
ax.minorticks_on()
ax.tick_params(which="minor", length=2, width=0.8)
# Add legends with transparent background
ax.legend(loc='upper left', fontsize=8, frameon=True, framealpha=0.0)
# Adjust layout and display the plot
plt.tight_layout()
plt.show()
Bar Chart
A bar chart is a graphical representation used to compare quantities of different categories or groups. Each bar’s height (or length) represents the value of the associated category, making it ideal for visualizing discrete data or summarizing datasets across distinct categories.
In scientific research, bar charts are widely used for comparing experimental results, analyzing trends in categorical data, and visualizing distributions of quantities across various groups or systems.
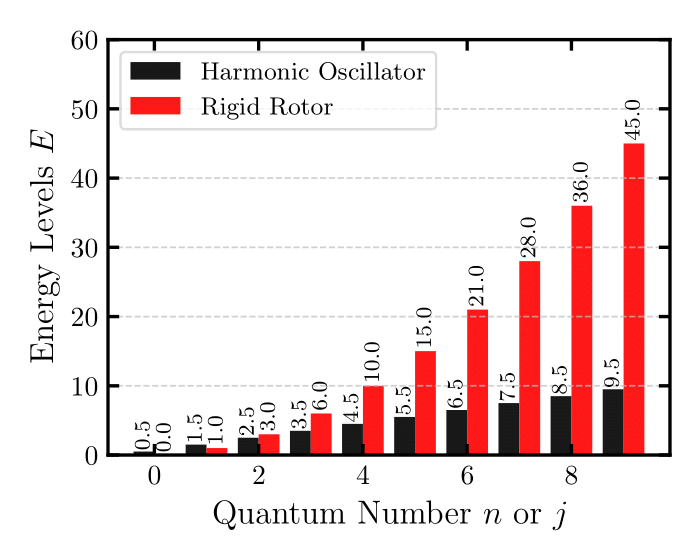
Python Code:
The code below generates a bar chart comparing energy eigenvalues for two quantum systems: a quantum harmonic oscillator (\(E_n = \hbar \omega (n + 1/2)\)) and a rigid rotor (\(E_j = \frac{\hbar^2 j(j+1)}{2I}\)). It employs professional styling suitable for publication, including LaTeX labels, compact figure dimensions, and staggered annotations for clarity.
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.pyplot import rcParams as r
# Configure plot aesthetics for Physical Review Letters-style formatting
r["figure.figsize"] = (3.5, 2.8) # Standard compact figure size
plt.rc("axes", linewidth=1.2)
plt.rcParams['text.usetex'] = True
plt.rcParams['font.family'] = 'serif'
plt.rcParams['font.serif'] = ['Computer Modern']
# Function to calculate energy levels for a quantum harmonic oscillator
def quantum_harmonic_energy_levels(n, h_bar=1, omega=1):
return h_bar * omega * (n + 0.5)
# Function to calculate energy levels for a rigid rotor
def rigid_rotor_energy_levels(j, h_bar=1, I=1):
return h_bar ** 2 * j * (j + 1) / (2 * I)
# Generate quantum numbers (n or j = 0 to 9)
quantum_numbers = np.arange(0, 10)
# Calculate energy levels for each system
harmonic_oscillator = [quantum_harmonic_energy_levels(n) for n in quantum_numbers]
rigid_rotor = [rigid_rotor_energy_levels(j) for j in quantum_numbers]
# Create a plot
fig, ax = plt.subplots()
width = 0.4 # Increased width of the bars
# Plot bars for each system
ax.bar(quantum_numbers - width / 2, harmonic_oscillator, width=width, color="black", label="Harmonic Oscillator", alpha=0.9)
ax.bar(quantum_numbers + width / 2, rigid_rotor, width=width, color="red", label="Rigid Rotor", alpha=0.9)
# Set axis labels and title
ax.set_xlabel(r"Quantum Number $n$ or $j$", fontsize=10)
ax.set_ylabel(r"Energy Levels $E$", fontsize=10)
# Configure ticks
ax.tick_params(which="both", direction="in", top=True, right=True, length=4, width=1.2, labelsize=10)
# Add grid for readability
ax.grid(axis='y', linestyle='--', linewidth=0.6, alpha=0.7)
# Add legend
ax.legend(fontsize=9, loc="upper left")
# Annotate energy values above the bars with staggered positioning to avoid overlap
for i, (ho, rr) in enumerate(zip(harmonic_oscillator, rigid_rotor)):
ax.text(i - width / 2, ho + 0.2, f"{ho:.1f}", ha='center', va='bottom', fontsize=8, rotation=90)
ax.text(i + width / 2, rr + 0.4, f"{rr:.1f}", ha='center', va='bottom', fontsize=8, rotation=90)
plt.ylim(0,60)
# Adjust layout and display the plot
plt.tight_layout()
plt.show()
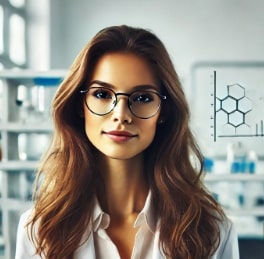
I am a science enthusiast with a keen interest in exploring the latest advancements in matter wave optics and related technologies.